Less, but better
Passwordless
Users can securely authorize through mail or phone. A verification code as well as a confirmation link will be sent to the user.
Seamless
Forms to integrate login and registration into any application without the need for any external redirects or dependencies.
Serverless
Let your serverless applications verify whether a request is authorized through webhooks.
Backendless
No private token or server required for authentication and registration. Webhooks can authorize users directly to an external service.
Anonymous Authentication
Create temporary accounts for instant access that can later be verified.
Privacy
None of your sensitive user information is exposed to iltio.
Client-Side Encryption
Allow your users to encrypt their data on the client safely.
Integrations
Authorize your Hasura applications using JSON Web Tokens instead of the webhook.
Fully Customizable Form
Login requires no redirects and the ready-made Open Source form plugin can be styled and seamlessly integrated into any application.
Secure Authentication
Registration and login both happen through entering a verification code or clicking a link sent to the user. You can specify which methods to allow users to authenticate with. Currently, mail address and phone number are supported.
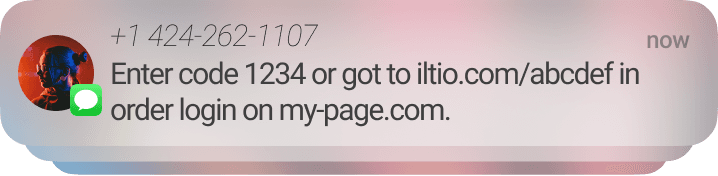
Simple HTTP Interface
If your framework isn't already supported it only takes a few requests to get authentication up and running.
Login / Registration
GET
https://iltio.com/api/
?name=MAIL/PHONE&token=APP_TOKEN
Client-Side Encryption
The iltio npm package provides helpers and components that allow you to prompt users to securely encrypt their data before sending it to your server.
import { encrypt, isEncrypted } from 'iltio'
import { Encryption } from 'iltio/react'
const PromptUserEncryption = () => !isEncrypted() && <Encryption />
const encryptedData = await encrypt(
{ id: 5, content: 'Hello World?' },
{ ignoreKeys: ['id'] },
)
Encryption • Client-Side • Privacy
Serverless Logging of Events
This service also allows you to add logs for events without the need for an additional backend server.
import { log } from 'iltio'
await log('My log message!', regularLogId)
await log(`Load page: ${page}`, trackPageLoadLogId)
await log(error.getMessage(), unexpectedErrorLogId)
Logs • Observability • Analytics